기본적인 canvas 그리기 및 스타일링
reference: pyplot 공식 Document 살펴보기
1 | import matplotlib.pyplot as plt |
1 | # plt.rcParams["figure.figsize"] = (12, 9) # figure size 설정 |
1. 밑 그림 그리기
1-1. 단일 그래프 (single graph)
plt.plot(df_name)
plt.show()
1 | ## data 생성 |
1-2. 다중 그래프 (multiple graphs)
- 여러 그래프를 같은 canvas 안에 그리기:
명령어plt.plot(df_name)
를 연속 사용- 새 그래프를 새로운 canvas 안에 그리기:
세 그래프를 그리기 전에plt.figure()
명령어를 추가
(1) 1개의 canvas 안에 다중 그래프 그리기
1 | data1 = np.arange(1, 51) |
(2) 새로운 canvas에서 새 그래프를 그리기
- figure()는 새로운 그래프 canvas를 생성한다
1 | data1 = np.arange(100, 201) |
1-3. 그래프 배열 (subplot / subplots)
여러 개 plot을 지정된 행,열수에 따라 배열해주기:
- plt.subplot(row, column, index) # 각 plot의 좌표 설정
- plt.subplots(행의 갯수, 열의 갯수) # 행,열수 설정
(1) subplot (plot의 좌표를 설정하기)
이 방법은 그래프마다 설정해줘야 함
plt.subplot(row, column, index) # 콤마를 제거해도 됨
1 | data1 = np.arange(100, 201) |
위의 코드와 동일하나, "콤마"를 제거한 상태
1 | data1 = np.arange(100, 201) |
1 | data1 = np.arange(100, 201) |
(2) subplots (배열 기준인 행,열수를 지정하기)
subplot와 다르게 subplots()명령어는 한번만 설정해주면 됨
plt.subplots(행의 갯수, 열의 갯수)
1 | data = np.arange(1, 51) |
2. 주요 스타일 옵션
1 | from IPython.display import Image |
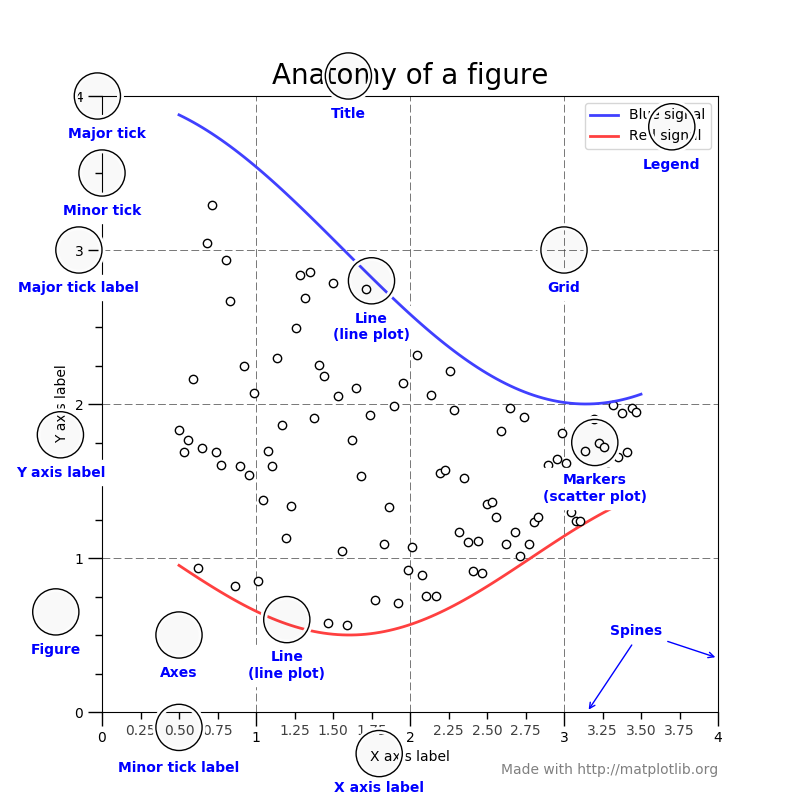
2-1. 타이틀
- 타이틀 추가: plt.title("…")
- 타이틀 fontsize 설정: plt.title("…", fontsize = … )
1 | plt.plot([1,2,3], [3,6,9]) |
Text(0.5, 1.0, '이것은 타이틀 입니다')
1 | plt.plot([1,2,3], [3,6,9]) |
Text(0.5, 1.0, '타이틀 fontsize를 키웁니다')
2-2. X, Y축 Label 설정
- plt.xlabel ( “x_name”, fontsize = …)
- plt.ylabel ( “y_name”, fontsize = …)
1 | plt.plot([1,2,3], [3,6,9]) |
Text(0, 0.5, 'Y축')
2-3. X, Y축 Tick 조정 (rotation)
Tick은 X, Y축에 위치한 눈금을 말한다
rotation 명령어를 통해 Tick의 각도를 조절할 수 있다
- plt.xticks ( rotation = … )
- plt.yticks ( rotation = … )
Rotation 각도는 역시개방향 회전각도를 말한다
1 | plt.plot(np.arange(10), np.arange(10)*2) |
D:\Anaconda\lib\site-packages\ipykernel_launcher.py:3: RuntimeWarning: divide by zero encountered in log
This is separate from the ipykernel package so we can avoid doing imports until
(array([-10., 0., 10., 20., 30., 40., 50., 60., 70., 80., 90.]),
<a list of 11 Text yticklabel objects>)
2-4. 범례 (Legend) 설정
plt.legend ( [ “name1” , “name2” , … ], fontsize = …)
1 | plt.plot(np.arange(10), np.arange(10)*2) |
D:\Anaconda\lib\site-packages\ipykernel_launcher.py:3: RuntimeWarning: divide by zero encountered in log
This is separate from the ipykernel package so we can avoid doing imports until
<matplotlib.legend.Legend at 0x173a5712888>
2-5. X와 Y의 한계점(Limit) 설정
- plt.xlim ( a, b )
- plt.ylim ( c, d )
1 | plt.plot(np.arange(10), np.arange(10)*2) |
D:\Anaconda\lib\site-packages\ipykernel_launcher.py:3: RuntimeWarning: divide by zero encountered in log
This is separate from the ipykernel package so we can avoid doing imports until
(0, 20)
2-6. 스타일 세부 설정 - 마커, 라인, 컬러
reference: 세부 Document 확인하기
스타일 세부 설정은 마커, 선의 동류 설정, 드리고 컬러가 있으며, 문다열로 세부설정을 하게 된다
(1) marker의 종류
- ‘.’ point marker
- ‘,’ pixel marker
- ‘o’ circle marker
- ‘v’ triangle_down marker
- ‘^’ triangle_up marker
- ‘<’ triangle_left marker
- ‘>’ triangle_right marker
- ‘1’ tri_down marker
- ‘2’ tri_up marker
- ‘3’ tri_left marker
- ‘4’ tri_right marker
- 's ’ square marker
- ‘p’ pentagon marker
- ‘*’ star marker
- ‘h’ hexagon1 marker
- ‘H’ hexagon2 marker
- ‘+’ plus marker
- ‘x’ x marker
- ‘D’ diamond marker
- ‘d’ thin_diamond marker
- ‘|’ vline marker
- ‘_’ hline marker
1 | # marker 스타일 설정 |
Text(0, 0.5, 'Y축')
(2) line의 종류
- ‘-’ solid line style
- ‘–’ dashed line style
- ‘-.’ dash-dot line style
- ‘:’ dotted line style
1 | # line 스타일 설정 |
Text(0, 0.5, 'Y축')
(3) color의 종류
- ‘b’ blue
- ‘g’ green
- ‘r’ red
- ‘c’ cyan
- ‘m’ magenta
- ‘y’ yellow
- ‘k’ black
- ‘w’ white
- more choices: matplotlib.colors (e.g. “purple”, “#008000”)
1 | # color 설정 |
Text(0, 0.5, 'Y축')
(4) Format: '[marker][line][color]'
example:
- ‘b’ # blue markers with default shape
- ‘or’ # red circles
- ‘-g’ # green solid line
- ‘–’ # dashed line with default color
- ‘^k:’ # black triangle_up markers connected by a dotted line
Each of them is optional. If not provided, the value from the style cycle is used. Exception: If line is given, but no marker, the data will be a line without markers.
1 | # "marker + line + color" format 설정 |
Text(0, 0.5, 'Y축')
(5) 색상 투명도 설정
- alpha = … (0.0 ~ 1.0)
1 | # color 투명도 설정 |
Text(0, 0.5, 'Y축')
2-7. 그리드 (grid) 설정
그리드 (grid) 추가: plt.grid()
1 | plt.plot(np.arange(10), np.arange(10)*2, marker='o', linestyle='-', color='b') |